# Quick Start
This walkthrough shows how to add Storyly to your Flutter application and show your first story in it.
You can also check out the demo on GitHub
Before you begin
This walkthrough contains sample instance information. However, if you want to work with your own content as well, please login into Storyly Dashboard (opens new window) and get your instance token.
The sample instance information for testing purposes;
eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJhY2NfaWQiOjc2MCwiYXBwX2lkIjo0MDUsImluc19pZCI6NDA0fQ.1AkqOy_lsiownTBNhVOUKc91uc9fDcAxfQZtpm3nj40
# Installation
Storyly Flutter package is available through pub.dev (opens new window). Source code is also available through the Storyly Flutter Github page. (opens new window), contributions are more than welcomed 🙂
Please check the Installing tab of Storyly Flutter's pub.dev page (opens new window) for installing steps.
# Android Installation
Storyly SDK is developed using Kotlin language. So, there are some requirements to use Kotlin plugin in the project. Especially if you're encountering a Plugin with id 'kotlin-android' not found.
error, this part fixes that.
You need to add kotlin-gradle-plugin
to Android project's main Gradle file (android/build.gradle
)
buildscript {
dependencies {
classpath("org.jetbrains.kotlin:kotlin-gradle-plugin:1.4.30")
}
}
Moreover, Storyly SDK is developed using the AndroidX library package instead of the Support Library. So, you need to ensure that your project also supports these packages. You can do this check and related lines if it's missing in the application's Android project's Gradle properties file (android/gradle.properties
)
android.useAndroidX=true
android.enableJetifier=true
WARNING
Although Storyly SDK targets Android API level 17 (Android 4.2, Jelly Bean)
or higher, PlatformView requires minimum API Android API level 20 (Android 4.4W, KitKat)
. So, you need to update your app's minimum SDK version to 20.
WARNING
Storyly SDK uses Material Design Components
. So, you need to update your dependencies accordingly. You can check the official documentation for Enabling Material Components (opens new window).
WARNING
If your app only targets Android 21 or higher (minSdkVersion) then multidex is already enabled by default and you do not need the multidex support library.
However, if your minSdkVersion is set to 20 or lower, then you must use the multidex support library and make the following modifications to your app project:
android {
defaultConfig {
// ...
multiDexEnabled true
// ...
}
}
dependencies {
implementation 'com.android.support:multidex:2.0.1'
}
# iOS Installation
First of all, you need to update pods of the app; please do not forget to run pod update
.
// this should be executed inside 'ios' folder
pod update
PlatformView's usage requires some updates on Info.plist
, add following lines:
<key>io.flutter.embedded_views_preview</key>
<true/>
How to edit Info.plist
In Xcode (or any editor), select the Info.plist from the Project Navigator. Right-click Open as -> Source Code. Now you will be able to add those two lines.
WARNING
Storyly SDK targets iOS 9
or higher.
WARNING
Please note that CocoaPods (opens new window) version should be 1.9+
# Import Storyly
Importing Storyly in your Flutter application is quite easy:
import 'package:storyly_flutter/storyly_flutter.dart';
# Initialize StorylyView
Storyly
extends Widget
so that you can use inherited functionality as it is. You can add the following lines inside any view of your app;
Container(
height: 120,
child: StorylyView(
onStorylyViewCreated: onStorylyViewCreated,
androidParam: StorylyParam()
..storylyId = STORYLY_INSTANCE_TOKEN,
iosParam: StorylyParam()
..storylyId = STORYLY_INSTANCE_TOKEN
),
)
void onStorylyViewCreated(StorylyViewController storylyViewController) {
this.storylyViewController = storylyViewController;
// You can call any function after this via StorylyViewController instance.
}
WARNING
storylyId : It's required for your app's correct initialization.
TIP
Please do not forget to use your own token. You can get your token from the Storyly Dashboard -> Settings -> App Settings (opens new window)
Just hit the run. Now, you should be able to enjoy Storyly
🎉!
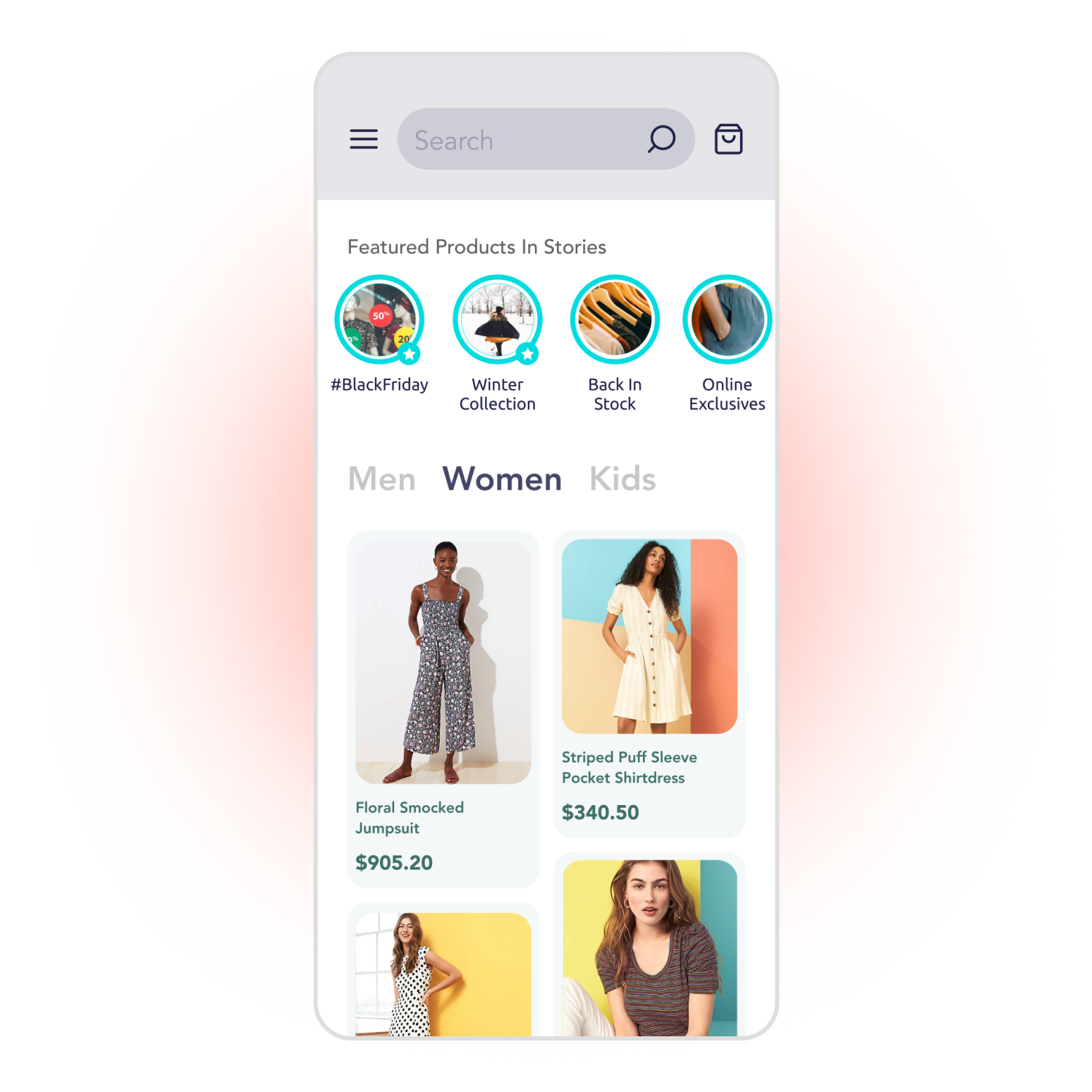
WARNING
If you can't see Storlyly in your application, please check that your token is correct. For more details please check console logs.